Qualified Export
Any package that is exported by a module is readable by any module that requires its module. However, sometimes it is necessary that only certain modules can access an exported package. This can be achieved by using the exports-to directive, as shown below at (1), where packageOne in moduleX is only exported to moduleA and moduleB:
module moduleX {
exports packageOne to moduleA, moduleB; // (1) Qualified export.
exports packageTwo; // (2) Unqualified export.
}
This is known as qualified export. Apart from moduleA and moduleB, no other module can access packageOne in moduleX. The compiler will issue an error if an attempt is made by any other module. Qualified export does not prevent the modules in the to clause to access other packages that are exported. Unqualified export of packageTwo, shown at (2), allows any module that requires moduleX to access packageTwo. Qualified export should be used judiciously, as its usage results in tight coupling between modules that can increase the degree of dependency between modules—a hallmark of poor software design.
Figure 19.7 shows how the module declaration of the factory module that uses the exports-to directive allows its package com.abc.factory to be accessible only by the seller and the client module. Any other module that requires module factory will not be able to access the com.abc.factory package in the factory module.
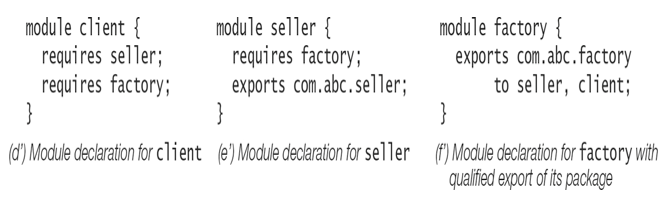
Figure 19.7 Qualified Export
19.4 Overview of Module Directives
An overview of all module directives is given in Table 19.2, together with references to where they are covered in this chapter.
Table 19.2 Overview of Module Directives
Directive | Description |
requires module (p. 1170) | The current module specifies a dependence on the specified module, allowing the current module to access exported public types in the specified module. |
requires transitive module (p. 1175) | Any module that requires the current module will have an implicit dependence on the specified module, and can access exported public types in the specified module on par with the current module. |
requires static module | The current module has a mandatory dependence on the specified module at compile time, but is optional at runtime. This is known as optional dependency. It is not discussed in this book. |
exports package (p. 1170) | The specified package exported by the current module is available to other modules that require the current module—that is, they can access public types in the specified package. This is called an unqualified export. |
exports package to module1, … (p. 1176) | The specified package exported by the current module is solely available to the modules specified in the to clause. That is, only those modules in the to clause that require the current module can access public types in the specified package. This is called a qualified export. |
opens package (p. 1191) | Access to the specified package is only granted through reflection (§25.6, p. 1587) at runtime for code in other modules. This is called an unqualified opens directive. |
opens package to module1, … (p. 1195) | Access to the specified package is only granted through reflection at runtime solely for code in modules specified in the to clause. This is called a qualified opens directive. |
provides type with type1, … (p. 1196) | The current module specifies that it provides a service (specified by type) that is implemented by one or more service providers (type1, … in the with clause implement the service). The current module is said to be a service provider. The service provided can be consumed by other modules that want to use this service. |
uses type (p. 1196) | The current module specifies that it uses a service (specified by type) and that a service provider that implements it may be discoverable at runtime. Typically, the current module is said to be a service locator, but can also be a service consumer if the locator is merged with the consumer. |
Modules add 10 restricted keywords to the language: exports, module, open, opens, provides, requires, to, transitive, uses, and with. The words open (see Table 19.3) and module are keywords only in the header of a module declaration, and the remaining in a particular context of a specific directive. The word static is already a keyword, but has a different meaning in the requires static directive.
Table 19.3 The open Modifier for Modules
Modifier | Description |
open module module { … } (p. 1196) | Unrestricted access to all packages in the module is granted through reflection at runtime for code in other modules. |
Leave a Reply